Gnome-Disks -- Take Ownership
Contents
Like most of the actions gnome-disks can perform on your storage devices TakeOwnership()
is also provided
by udisks. TakeOwnership()
changes the ownership of the filesystem to the user (UID) and group (GID) of the calling user.
It is possible to call this method with the recursive mode to change the ownership for all subdirectories and files.
The operation is not available on fat and ntfs only for the typical unix filesystems.
Merge Requests: #48 #51
Documentation: TakeOwnership()
Repositories: gnome-disks | udisks
Basic Implementation
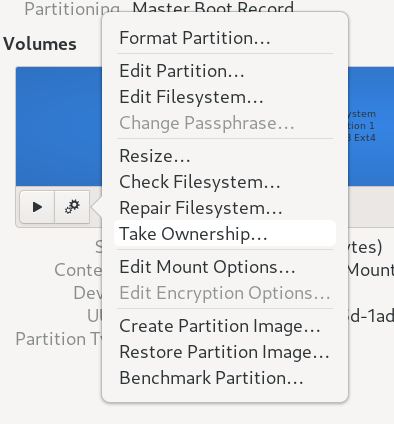
The first step was to add a new button at the appropriate position.
The volume menu contains already some similar actions like Repair Filesystem and Check Filesystem so Take Ownership would fit perfectly.
To change the layout I had to edit src/disks/ui/volume-menu.ui
and add a new item right below the Repair Filesystem item.
The merge request
includes a list of all the changes.
TakeOwnership()
does not work for drives with a ntfs or fat filesystem so the best solution was to only enable
the action of the button with g_simple_action_set_enabled ()
when the type of the filesystem is not fat or ntfs.
I created a new function and mapped it to the button. Now every time the button gets
pressed on_volume_menu_item_take_ownership ()
gets called with our selected filesystem. When the operation is finished
a callback function gets called and throws an error message if the operation was not successful.
Here is the C code of the two new functions:
|
|
Confirmation Dialog
All the other actions in the volume menu have a confirmation dialog with a basic description,
so the Take Ownership action should also have one. This dialog will also contain a check button to
enable and disable the recursive mode.
To archive this, I created a new file src/disks/ui/take-ownership-dialog.ui
.
The file
contains all the information to define the visual of a confirmation dialog with a description, and a check button
for the recursion mode.
I also had to change the code from earlier a little. The fs_take_ownership_cb ()
now creates our message dialog with
GtkBuilder and gtk_builder_new_from_resource ()
.
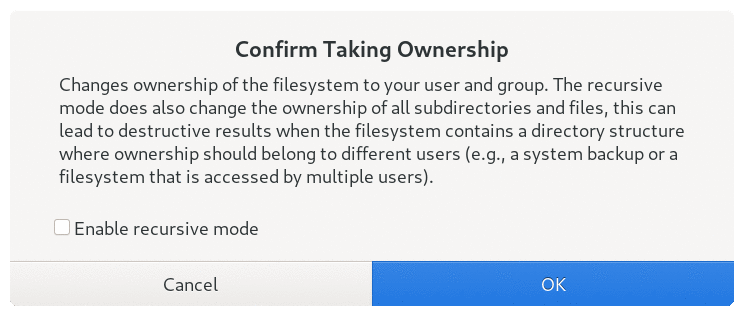
The recursive mode is a potential dangerous operation, so the ok button should get the destructive-action
style
to visually warn the user by turning red.
To detect a button press, we have to connect to the toogled signal of the check button with g_signal_connect ()
.
Now we can change the style according to the state of the button.
Special thanks to Kai Lüke — the maintainer of gnome-disks — for giving me feedback and helping me to implement this feature.
If you want to learn more about programming for gnome — check out gtk or the gnome developer center.
Author Manuel Wassermann
LastMod 2021-07-18